Note
Go to the end to download the full example code.
Lecture 2: Gym
# # Lecture 2: Gym
Dependencies and setup
(this can take a minute or so…)
# !pip install swig
# !pip install rldurham # latest release
# !pip install git+https://github.com/robert-lieck/rldurham.git@main # latest main version (typically same as release)
# !pip install git+https://github.com/robert-lieck/rldurham.git@dev # latest dev version
import gymnasium as gym
import rldurham as rld # Reinforcement Learning Durham package with helper functions
Basic environment
env = gym.make('CartPole-v1', render_mode="human")
observation, info = env.reset(seed=42)
for episode in range(10):
observation, info = env.reset()
done = False
while not done:
action = env.action_space.sample() # random action
observation, reward, terminated, truncated, info = env.step(action)
done = terminated or truncated
env.close()
Reinforcement Learning Durham:
_rldurham_ Python package
# drop-in for gym.make that enables logging (use render_mode="rgb_array" to enable video rendering)
env = rld.make('CartPole-v1', render_mode="rgb_array")
# record statistics (returned in info) and videos
env = rld.Recorder(
env,
smoothing=10, # track rolling averages (not required for coursework)
video=True, # enable recording videos
video_folder="videos", # folder for videos
video_prefix="xxxx00-agent-video", # prefix for videos
logs=True, # keep logs
)
# make reproducible by seeding everything (python, numpy, pytorch, env)
# this also calls env.reset
seed, observation, info = rld.seed_everything(42, env)
# optionally track statistics for plotting
tracker = rld.InfoTracker()
# run episodes
for episode in range(11):
# recording statistics and video can be switched on and off (video recording is slow!)
env.info = episode % 2 == 0 # track every other episode (usually tracking every episode is fine)
env.video = episode % 4 == 0 # you only want to record videos every x episodes (set BEFORE calling reset!)
#######################################################################
# this is the same as above
observation, info = env.reset()
done = False
while not done:
action = env.action_space.sample() # Random action
observation, reward, terminated, truncated, info = env.step(action)
done = terminated or truncated
#######################################################################
if done:
# per-episode statistics are returned by Recorder wrapper in info
# 'idx': index/count of the episode
# 'length': length of the episode
# 'r_sum': sum of rewards in episode
# 'r_mean': mean of rewards in episode
# 'r_std': standard deviation of rewards in episode
# 'length_': average `length' over smoothing window
# 'r_sum_': reward sum over smoothing window (not the average)
# 'r_mean_': average reward sum per episode over smoothing window (i.e. average of `r_sum')
# 'r_std_': standard deviation of reward sum per episode over smoothing window
# InfoTracker turns these into arrays over time
tracker.track(info)
print(tracker.info)
# some plotting functionality is provided (this will refresh in notebooks)
# - combinations of ``r_mean`` and ``r_std`` or ``r_mean_`` and ``r_std_`` are most insightful
# - for `CartPole`, ``length`` and ``r_sum`` are the same as there is a unit reward for each
# time step of successful balancing
tracker.plot(r_mean_=True, r_std_=True,
length=dict(linestyle='--', marker='o'),
r_sum=dict(linestyle='', marker='x'))
# don't forget to close environment (e.g. triggers last video save)
env.close()
# write log file (for coursework)
env.write_log(folder="logs", file="xxxx00-agent-log.txt")
/home/runner/.local/lib/python3.12/site-packages/gymnasium/wrappers/rendering.py:283: UserWarning: WARN: Overwriting existing videos at /home/runner/work/rldurham/rldurham/examples/videos folder (try specifying a different `video_folder` for the `RecordVideo` wrapper if this is not desired)
logger.warn(
{'recorder': {'idx': [0], 'length': [29], 'r_sum': [29.0], 'r_mean': [1.0], 'r_std': [0.0], 'length_': [29.0], 'r_sum_': [29.0], 'r_mean_': [29.0], 'r_std_': [0.0]}}
{'recorder': {'idx': [0], 'length': [29], 'r_sum': [29.0], 'r_mean': [1.0], 'r_std': [0.0], 'length_': [29.0], 'r_sum_': [29.0], 'r_mean_': [29.0], 'r_std_': [0.0]}}
{'recorder': {'idx': [0, 2], 'length': [29, 69], 'r_sum': [29.0, 69.0], 'r_mean': [1.0, 1.0], 'r_std': [0.0, 0.0], 'length_': [29.0, 49.0], 'r_sum_': [29.0, 98.0], 'r_mean_': [29.0, 49.0], 'r_std_': [0.0, 20.0]}}
{'recorder': {'idx': [0, 2], 'length': [29, 69], 'r_sum': [29.0, 69.0], 'r_mean': [1.0, 1.0], 'r_std': [0.0, 0.0], 'length_': [29.0, 49.0], 'r_sum_': [29.0, 98.0], 'r_mean_': [29.0, 49.0], 'r_std_': [0.0, 20.0]}}
{'recorder': {'idx': [0, 2, 4], 'length': [29, 69, 39], 'r_sum': [29.0, 69.0, 39.0], 'r_mean': [1.0, 1.0, 1.0], 'r_std': [0.0, 0.0, 0.0], 'length_': [29.0, 49.0, 45.666666666666664], 'r_sum_': [29.0, 98.0, 137.0], 'r_mean_': [29.0, 49.0, 45.666666666666664], 'r_std_': [0.0, 20.0, 16.996731711975958]}}
{'recorder': {'idx': [0, 2, 4], 'length': [29, 69, 39], 'r_sum': [29.0, 69.0, 39.0], 'r_mean': [1.0, 1.0, 1.0], 'r_std': [0.0, 0.0, 0.0], 'length_': [29.0, 49.0, 45.666666666666664], 'r_sum_': [29.0, 98.0, 137.0], 'r_mean_': [29.0, 49.0, 45.666666666666664], 'r_std_': [0.0, 20.0, 16.996731711975958]}}
{'recorder': {'idx': [0, 2, 4, 6], 'length': [29, 69, 39, 14], 'r_sum': [29.0, 69.0, 39.0, 14.0], 'r_mean': [1.0, 1.0, 1.0, 1.0], 'r_std': [0.0, 0.0, 0.0, 0.0], 'length_': [29.0, 49.0, 45.666666666666664, 37.75], 'r_sum_': [29.0, 98.0, 137.0, 151.0], 'r_mean_': [29.0, 49.0, 45.666666666666664, 37.75], 'r_std_': [0.0, 20.0, 16.996731711975958, 20.116846174288852]}}
{'recorder': {'idx': [0, 2, 4, 6], 'length': [29, 69, 39, 14], 'r_sum': [29.0, 69.0, 39.0, 14.0], 'r_mean': [1.0, 1.0, 1.0, 1.0], 'r_std': [0.0, 0.0, 0.0, 0.0], 'length_': [29.0, 49.0, 45.666666666666664, 37.75], 'r_sum_': [29.0, 98.0, 137.0, 151.0], 'r_mean_': [29.0, 49.0, 45.666666666666664, 37.75], 'r_std_': [0.0, 20.0, 16.996731711975958, 20.116846174288852]}}
{'recorder': {'idx': [0, 2, 4, 6, 8], 'length': [29, 69, 39, 14, 28], 'r_sum': [29.0, 69.0, 39.0, 14.0, 28.0], 'r_mean': [1.0, 1.0, 1.0, 1.0, 1.0], 'r_std': [0.0, 0.0, 0.0, 0.0, 0.0], 'length_': [29.0, 49.0, 45.666666666666664, 37.75, 35.8], 'r_sum_': [29.0, 98.0, 137.0, 151.0, 179.0], 'r_mean_': [29.0, 49.0, 45.666666666666664, 37.75, 35.8], 'r_std_': [0.0, 20.0, 16.996731711975958, 20.116846174288852, 18.410866356584094]}}
{'recorder': {'idx': [0, 2, 4, 6, 8], 'length': [29, 69, 39, 14, 28], 'r_sum': [29.0, 69.0, 39.0, 14.0, 28.0], 'r_mean': [1.0, 1.0, 1.0, 1.0, 1.0], 'r_std': [0.0, 0.0, 0.0, 0.0, 0.0], 'length_': [29.0, 49.0, 45.666666666666664, 37.75, 35.8], 'r_sum_': [29.0, 98.0, 137.0, 151.0, 179.0], 'r_mean_': [29.0, 49.0, 45.666666666666664, 37.75, 35.8], 'r_std_': [0.0, 20.0, 16.996731711975958, 20.116846174288852, 18.410866356584094]}}
{'recorder': {'idx': [0, 2, 4, 6, 8, 10], 'length': [29, 69, 39, 14, 28, 25], 'r_sum': [29.0, 69.0, 39.0, 14.0, 28.0, 25.0], 'r_mean': [1.0, 1.0, 1.0, 1.0, 1.0, 1.0], 'r_std': [0.0, 0.0, 0.0, 0.0, 0.0, 0.0], 'length_': [29.0, 49.0, 45.666666666666664, 37.75, 35.8, 34.0], 'r_sum_': [29.0, 98.0, 137.0, 151.0, 179.0, 204.0], 'r_mean_': [29.0, 49.0, 45.666666666666664, 37.75, 35.8, 34.0], 'r_std_': [0.0, 20.0, 16.996731711975958, 20.116846174288852, 18.410866356584094, 17.281975195754296]}}
# print log file
import pandas as pd
log_file = "logs/xxxx00-agent-log.txt"
print(f"log file: {log_file}")
print(pd.read_csv(log_file, sep="\t").head())
log file: logs/xxxx00-agent-log.txt
count reward_sum squared_reward_sum length
0 0 29.0 29.0 29
1 1 17.0 17.0 17
2 2 69.0 69.0 69
3 3 15.0 15.0 15
4 4 39.0 39.0 39
# show video
import os, re
from ipywidgets import Video
video_file = "videos/" + sorted(f for f in os.listdir("videos") if re.match(r".+episode=4.+\.mp4", f))[0]
print(f"video file: {video_file}")
Video.from_file(video_file)
video file: videos/xxxx00-agent-video,episode=4,score=39.0.mp4
Video(value=b'\x00\x00\x00 ftypisom\x00\x00\x02\x00isomiso2avc1mp41\x00\x00\x00\x08free...')
Different environments
## render mode
# rm = "human" # for rendering in separate window
rm = 'rgb_array' # for recording videos or rendering in notebook
# rm = None # no rendering
## select environment
env = rld.make('CartPole-v1', render_mode=rm) # easy discrete
# env = rld.make('FrozenLake-v1', render_mode=rm) # easy discrete
# env = rld.make('LunarLander-v3', render_mode=rm) # discrete
# env = rld.make('ALE/Breakout-v5', render_mode=rm) # discrete (atari)
# env = rld.make('Pong-ram-v4', render_mode=rm) # discrete (atari)
# env = rld.make('Gravitar-ram-v4', render_mode=rm) # hard discrete (atari)
#
# env = rld.make('Pendulum-v1', render_mode=rm) # easy continuous
# env = rld.make('LunarLanderContinuous-v3', render_mode=rm) # continuous
# env = rld.make('BipedalWalker-v3', render_mode=rm) # continuous
# env = rld.make('BipedalWalkerHardcore-v3', render_mode=rm) # hard continuous
## wrap for stats and video recording (requires render_mode='rgb_array')
# env = rld.Recorder(env, video=True)
# env.video = False # deactivate
## there are many more!
# gym.pprint_registry()
# get some info
discrete_act = hasattr(env.action_space, 'n')
discrete_obs = hasattr(env.observation_space, 'n')
act_dim = env.action_space.n if discrete_act else env.action_space.shape[0]
obs_dim = env.observation_space.n if discrete_obs else env.observation_space.shape[0]
discrete_act, discrete_obs, act_dim, obs_dim = rld.env_info(env) # same
print(f"The environment has "
f"{act_dim}{(f' discrete' if discrete_act else f'-dimensional continuous')} actions and "
f"{obs_dim}{(f' discrete' if discrete_obs else f'-dimensional continuous')} observations.")
print('The maximum timesteps is: {}'.format(env.spec.max_episode_steps))
The environment has 2 discrete actions and 4-dimensional continuous observations.
The maximum timesteps is: 500
for episode in range(1):
observation, info = env.reset()
rld.render(env, clear=True)
done = False
for step in range(200):
action = env.action_space.sample() # random action
observation, reward, terminated, truncated, info = env.step(action)
rld.render(env, clear=True)
done = terminated or truncated
if done:
break
env.close()
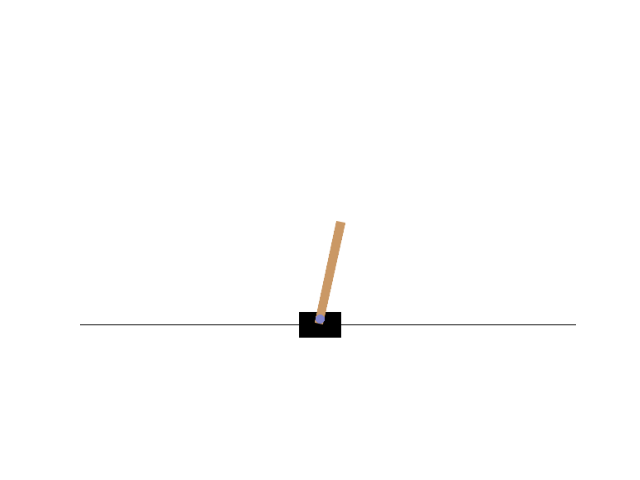
Training an agent
import numpy as np
import torch
device = torch.device('cuda') if torch.cuda.is_available() else torch.device('cpu')
print('The device is: {}'.format(device))
if device.type != 'cpu': print('It\'s recommended to train on the cpu for this')
The device is: cpu
class Agent(torch.nn.Module):
def __init__(self, env):
super().__init__()
self.discrete_act, self.discrete_obs, self.act_dim, self.obs_dim = rld.env_info(env)
def prob_action(self, obs):
return np.ones(self.act_dim)/self.act_dim
def sample_action(self, prob):
if self.discrete_act:
return np.random.choice(self.act_dim, p=prob)
else:
return np.random.uniform(-1.0, 1.0, size=self.act_dim)
def train(self):
return
def put_data(self, item):
return
# init environment
env = rld.make('CartPole-v1')
env = rld.Recorder(env, smoothing=10)
# seed
rld.seed_everything(seed=42, env=env)
# init agent
agent = Agent(env)
# training procedure
tracker = rld.InfoTracker()
for episode in range(201):
obs, info = env.reset()
done = False
# get episode
while not done:
# select action
prob = agent.prob_action(obs)
action = agent.sample_action(prob)
# take action in environment
next_obs, reward, terminated, truncated, info = env.step(action)
done = terminated or truncated
agent.put_data((reward, prob[action] if agent.discrete_act else None))
obs = next_obs
# track and plot
tracker.track(info)
if episode % 10 == 0:
tracker.plot(r_mean_=True, r_std_=True)
# update agent's policy
agent.train()
env.close()
/home/runner/work/rldurham/rldurham/rldurham/__init__.py:450: RuntimeWarning: More than 20 figures have been opened. Figures created through the pyplot interface (`matplotlib.pyplot.figure`) are retained until explicitly closed and may consume too much memory. (To control this warning, see the rcParam `figure.max_open_warning`). Consider using `matplotlib.pyplot.close()`.
fig, ax = plt.subplots(1, 1)
REINFORCE agent example
This code is based on: https://github.com/seungeunrho/minimalRL
Note: these implementations are good to study, although most are for discrete action spaces
# this is an implementation of REINFORCE (taught in lecture 8) - one of the simplest classical policy gradient methods
# this will only work for simple discrete control problems like cart pole or (slowly) lunar lander discrete
plot_interval = 50
video_every = 500
max_episodes = 5000
class Agent(torch.nn.Module):
def __init__(self, env):
super().__init__()
discrete_act, discrete_obs, act_dim, obs_dim = rld.env_info(env)
if not discrete_act:
raise RuntimeError("REINFORCE only works for discrete action spaces")
self.data = []
self.fc1 = torch.nn.Linear(obs_dim, 128)
self.fc2 = torch.nn.Linear(128, act_dim)
self.optimizer = torch.optim.Adam(self.parameters(), lr=0.0002)
def sample_action(self, prob):
m = torch.Categorical(prob)
a = m.sample()
return a.item()
def prob_action(self, s):
x = torch.F.relu(self.fc1(torch.from_numpy(s).float()))
return torch.F.softmax(self.fc2(x), dim=0)
def put_data(self, item):
self.data.append(item)
def train(self):
R = 0
self.optimizer.zero_grad()
for r, prob in self.data[::-1]:
R = r + 0.98 * R
loss = -torch.log(prob) * R
loss.backward()
self.optimizer.step()
self.data = []
Custom environments (here: multi-armed bandits)
# one armed bandits are slot machines where you can win or loose money
# some casinos tune them, so some machines are less successful (the probability distribution of winning)
# the machines also dish out varying rewards (the reward distribution)
# so basically each machine has some probability of dishing out £ reward (p_dist doesn't need to sum to 1) else it gives £0
class BanditEnv(gym.Env):
def __init__(self, p_dist=[0.4,0.2,0.1,0.1,0.1,0.7], r_dist=[1,0.1,2,0.5,6,70]):
self.p_dist = p_dist
self.r_dist = r_dist
self.n_bandits = len(p_dist)
self.action_space = gym.spaces.Discrete(self.n_bandits)
self.observation_space = gym.spaces.Discrete(1)
def step(self, action):
assert self.action_space.contains(action)
reward = -25
terminated = True
truncated = False
if np.random.uniform() < self.p_dist[action]:
if not isinstance(self.r_dist[action], list):
reward += self.r_dist[action]
else:
reward += np.random.normal(self.r_dist[action][0], self.r_dist[action][1])
return np.zeros(1), reward, terminated, truncated, {}
def reset(self, seed=None, options=None):
if seed is not None:
self.np_random, seed = gym.utils.seeding.np_random(seed)
return np.zeros(1), {}
def render(self):
pass
# initialise
discrete = True
env = BanditEnv()
obs_dim = 1
act_dim = len(env.p_dist)
env.spec = gym.envs.registration.EnvSpec('BanditEnv-v0', max_episode_steps=5)
max_episodes = 1000
for episode in range(10):
observation, info = env.reset()
done = False
while not done:
action = env.action_space.sample() # random action
observation, reward, terminated, truncated, info = env.step(action)
print(action, reward)
done = terminated or truncated
env.close()
2 -25
5 45
0 -24
2 -25
3 -25
3 -25
5 45
5 45
4 -25
0 -25
gym.register(id="BanditEnv-v0", entry_point=BanditEnv)
gym.pprint_registry()
===== classic_control =====
Acrobot-v1 CartPole-v0 CartPole-v1
MountainCar-v0 MountainCarContinuous-v0 Pendulum-v1
===== phys2d =====
phys2d/CartPole-v0 phys2d/CartPole-v1 phys2d/Pendulum-v0
===== box2d =====
BipedalWalker-v3 BipedalWalkerHardcore-v3 CarRacing-v3
LunarLander-v3 LunarLanderContinuous-v3
===== toy_text =====
Blackjack-v1 CliffWalking-v0 FrozenLake-v1
FrozenLake8x8-v1 Taxi-v3
===== tabular =====
tabular/Blackjack-v0 tabular/CliffWalking-v0
===== mujoco =====
Ant-v2 Ant-v3 Ant-v4
Ant-v5 HalfCheetah-v2 HalfCheetah-v3
HalfCheetah-v4 HalfCheetah-v5 Hopper-v2
Hopper-v3 Hopper-v4 Hopper-v5
Humanoid-v2 Humanoid-v3 Humanoid-v4
Humanoid-v5 HumanoidStandup-v2 HumanoidStandup-v4
HumanoidStandup-v5 InvertedDoublePendulum-v2 InvertedDoublePendulum-v4
InvertedDoublePendulum-v5 InvertedPendulum-v2 InvertedPendulum-v4
InvertedPendulum-v5 Pusher-v2 Pusher-v4
Pusher-v5 Reacher-v2 Reacher-v4
Reacher-v5 Swimmer-v2 Swimmer-v3
Swimmer-v4 Swimmer-v5 Walker2d-v2
Walker2d-v3 Walker2d-v4 Walker2d-v5
===== None =====
BanditEnv-v0 GymV21Environment-v0 GymV26Environment-v0
===== env =====
Adventure-ram-v0 Adventure-ram-v4 Adventure-ramDeterministic-v0
Adventure-ramDeterministic-v4 Adventure-ramNoFrameskip-v0 Adventure-ramNoFrameskip-v4
Adventure-v0 Adventure-v4 AdventureDeterministic-v0
AdventureDeterministic-v4 AdventureNoFrameskip-v0 AdventureNoFrameskip-v4
AirRaid-ram-v0 AirRaid-ram-v4 AirRaid-ramDeterministic-v0
AirRaid-ramDeterministic-v4 AirRaid-ramNoFrameskip-v0 AirRaid-ramNoFrameskip-v4
AirRaid-v0 AirRaid-v4 AirRaidDeterministic-v0
AirRaidDeterministic-v4 AirRaidNoFrameskip-v0 AirRaidNoFrameskip-v4
Alien-ram-v0 Alien-ram-v4 Alien-ramDeterministic-v0
Alien-ramDeterministic-v4 Alien-ramNoFrameskip-v0 Alien-ramNoFrameskip-v4
Alien-v0 Alien-v4 AlienDeterministic-v0
AlienDeterministic-v4 AlienNoFrameskip-v0 AlienNoFrameskip-v4
Amidar-ram-v0 Amidar-ram-v4 Amidar-ramDeterministic-v0
Amidar-ramDeterministic-v4 Amidar-ramNoFrameskip-v0 Amidar-ramNoFrameskip-v4
Amidar-v0 Amidar-v4 AmidarDeterministic-v0
AmidarDeterministic-v4 AmidarNoFrameskip-v0 AmidarNoFrameskip-v4
Assault-ram-v0 Assault-ram-v4 Assault-ramDeterministic-v0
Assault-ramDeterministic-v4 Assault-ramNoFrameskip-v0 Assault-ramNoFrameskip-v4
Assault-v0 Assault-v4 AssaultDeterministic-v0
AssaultDeterministic-v4 AssaultNoFrameskip-v0 AssaultNoFrameskip-v4
Asterix-ram-v0 Asterix-ram-v4 Asterix-ramDeterministic-v0
Asterix-ramDeterministic-v4 Asterix-ramNoFrameskip-v0 Asterix-ramNoFrameskip-v4
Asterix-v0 Asterix-v4 AsterixDeterministic-v0
AsterixDeterministic-v4 AsterixNoFrameskip-v0 AsterixNoFrameskip-v4
Asteroids-ram-v0 Asteroids-ram-v4 Asteroids-ramDeterministic-v0
Asteroids-ramDeterministic-v4 Asteroids-ramNoFrameskip-v0 Asteroids-ramNoFrameskip-v4
Asteroids-v0 Asteroids-v4 AsteroidsDeterministic-v0
AsteroidsDeterministic-v4 AsteroidsNoFrameskip-v0 AsteroidsNoFrameskip-v4
Atlantis-ram-v0 Atlantis-ram-v4 Atlantis-ramDeterministic-v0
Atlantis-ramDeterministic-v4 Atlantis-ramNoFrameskip-v0 Atlantis-ramNoFrameskip-v4
Atlantis-v0 Atlantis-v4 AtlantisDeterministic-v0
AtlantisDeterministic-v4 AtlantisNoFrameskip-v0 AtlantisNoFrameskip-v4
BankHeist-ram-v0 BankHeist-ram-v4 BankHeist-ramDeterministic-v0
BankHeist-ramDeterministic-v4 BankHeist-ramNoFrameskip-v0 BankHeist-ramNoFrameskip-v4
BankHeist-v0 BankHeist-v4 BankHeistDeterministic-v0
BankHeistDeterministic-v4 BankHeistNoFrameskip-v0 BankHeistNoFrameskip-v4
BattleZone-ram-v0 BattleZone-ram-v4 BattleZone-ramDeterministic-v0
BattleZone-ramDeterministic-v4 BattleZone-ramNoFrameskip-v0 BattleZone-ramNoFrameskip-v4
BattleZone-v0 BattleZone-v4 BattleZoneDeterministic-v0
BattleZoneDeterministic-v4 BattleZoneNoFrameskip-v0 BattleZoneNoFrameskip-v4
BeamRider-ram-v0 BeamRider-ram-v4 BeamRider-ramDeterministic-v0
BeamRider-ramDeterministic-v4 BeamRider-ramNoFrameskip-v0 BeamRider-ramNoFrameskip-v4
BeamRider-v0 BeamRider-v4 BeamRiderDeterministic-v0
BeamRiderDeterministic-v4 BeamRiderNoFrameskip-v0 BeamRiderNoFrameskip-v4
Berzerk-ram-v0 Berzerk-ram-v4 Berzerk-ramDeterministic-v0
Berzerk-ramDeterministic-v4 Berzerk-ramNoFrameskip-v0 Berzerk-ramNoFrameskip-v4
Berzerk-v0 Berzerk-v4 BerzerkDeterministic-v0
BerzerkDeterministic-v4 BerzerkNoFrameskip-v0 BerzerkNoFrameskip-v4
Bowling-ram-v0 Bowling-ram-v4 Bowling-ramDeterministic-v0
Bowling-ramDeterministic-v4 Bowling-ramNoFrameskip-v0 Bowling-ramNoFrameskip-v4
Bowling-v0 Bowling-v4 BowlingDeterministic-v0
BowlingDeterministic-v4 BowlingNoFrameskip-v0 BowlingNoFrameskip-v4
Boxing-ram-v0 Boxing-ram-v4 Boxing-ramDeterministic-v0
Boxing-ramDeterministic-v4 Boxing-ramNoFrameskip-v0 Boxing-ramNoFrameskip-v4
Boxing-v0 Boxing-v4 BoxingDeterministic-v0
BoxingDeterministic-v4 BoxingNoFrameskip-v0 BoxingNoFrameskip-v4
Breakout-ram-v0 Breakout-ram-v4 Breakout-ramDeterministic-v0
Breakout-ramDeterministic-v4 Breakout-ramNoFrameskip-v0 Breakout-ramNoFrameskip-v4
Breakout-v0 Breakout-v4 BreakoutDeterministic-v0
BreakoutDeterministic-v4 BreakoutNoFrameskip-v0 BreakoutNoFrameskip-v4
Carnival-ram-v0 Carnival-ram-v4 Carnival-ramDeterministic-v0
Carnival-ramDeterministic-v4 Carnival-ramNoFrameskip-v0 Carnival-ramNoFrameskip-v4
Carnival-v0 Carnival-v4 CarnivalDeterministic-v0
CarnivalDeterministic-v4 CarnivalNoFrameskip-v0 CarnivalNoFrameskip-v4
Centipede-ram-v0 Centipede-ram-v4 Centipede-ramDeterministic-v0
Centipede-ramDeterministic-v4 Centipede-ramNoFrameskip-v0 Centipede-ramNoFrameskip-v4
Centipede-v0 Centipede-v4 CentipedeDeterministic-v0
CentipedeDeterministic-v4 CentipedeNoFrameskip-v0 CentipedeNoFrameskip-v4
ChopperCommand-ram-v0 ChopperCommand-ram-v4 ChopperCommand-ramDeterministic-v0
ChopperCommand-ramDeterministic-v4 ChopperCommand-ramNoFrameskip-v0 ChopperCommand-ramNoFrameskip-v4
ChopperCommand-v0 ChopperCommand-v4 ChopperCommandDeterministic-v0
ChopperCommandDeterministic-v4 ChopperCommandNoFrameskip-v0 ChopperCommandNoFrameskip-v4
CrazyClimber-ram-v0 CrazyClimber-ram-v4 CrazyClimber-ramDeterministic-v0
CrazyClimber-ramDeterministic-v4 CrazyClimber-ramNoFrameskip-v0 CrazyClimber-ramNoFrameskip-v4
CrazyClimber-v0 CrazyClimber-v4 CrazyClimberDeterministic-v0
CrazyClimberDeterministic-v4 CrazyClimberNoFrameskip-v0 CrazyClimberNoFrameskip-v4
Defender-ram-v0 Defender-ram-v4 Defender-ramDeterministic-v0
Defender-ramDeterministic-v4 Defender-ramNoFrameskip-v0 Defender-ramNoFrameskip-v4
Defender-v0 Defender-v4 DefenderDeterministic-v0
DefenderDeterministic-v4 DefenderNoFrameskip-v0 DefenderNoFrameskip-v4
DemonAttack-ram-v0 DemonAttack-ram-v4 DemonAttack-ramDeterministic-v0
DemonAttack-ramDeterministic-v4 DemonAttack-ramNoFrameskip-v0 DemonAttack-ramNoFrameskip-v4
DemonAttack-v0 DemonAttack-v4 DemonAttackDeterministic-v0
DemonAttackDeterministic-v4 DemonAttackNoFrameskip-v0 DemonAttackNoFrameskip-v4
DoubleDunk-ram-v0 DoubleDunk-ram-v4 DoubleDunk-ramDeterministic-v0
DoubleDunk-ramDeterministic-v4 DoubleDunk-ramNoFrameskip-v0 DoubleDunk-ramNoFrameskip-v4
DoubleDunk-v0 DoubleDunk-v4 DoubleDunkDeterministic-v0
DoubleDunkDeterministic-v4 DoubleDunkNoFrameskip-v0 DoubleDunkNoFrameskip-v4
ElevatorAction-ram-v0 ElevatorAction-ram-v4 ElevatorAction-ramDeterministic-v0
ElevatorAction-ramDeterministic-v4 ElevatorAction-ramNoFrameskip-v0 ElevatorAction-ramNoFrameskip-v4
ElevatorAction-v0 ElevatorAction-v4 ElevatorActionDeterministic-v0
ElevatorActionDeterministic-v4 ElevatorActionNoFrameskip-v0 ElevatorActionNoFrameskip-v4
Enduro-ram-v0 Enduro-ram-v4 Enduro-ramDeterministic-v0
Enduro-ramDeterministic-v4 Enduro-ramNoFrameskip-v0 Enduro-ramNoFrameskip-v4
Enduro-v0 Enduro-v4 EnduroDeterministic-v0
EnduroDeterministic-v4 EnduroNoFrameskip-v0 EnduroNoFrameskip-v4
FishingDerby-ram-v0 FishingDerby-ram-v4 FishingDerby-ramDeterministic-v0
FishingDerby-ramDeterministic-v4 FishingDerby-ramNoFrameskip-v0 FishingDerby-ramNoFrameskip-v4
FishingDerby-v0 FishingDerby-v4 FishingDerbyDeterministic-v0
FishingDerbyDeterministic-v4 FishingDerbyNoFrameskip-v0 FishingDerbyNoFrameskip-v4
Freeway-ram-v0 Freeway-ram-v4 Freeway-ramDeterministic-v0
Freeway-ramDeterministic-v4 Freeway-ramNoFrameskip-v0 Freeway-ramNoFrameskip-v4
Freeway-v0 Freeway-v4 FreewayDeterministic-v0
FreewayDeterministic-v4 FreewayNoFrameskip-v0 FreewayNoFrameskip-v4
Frostbite-ram-v0 Frostbite-ram-v4 Frostbite-ramDeterministic-v0
Frostbite-ramDeterministic-v4 Frostbite-ramNoFrameskip-v0 Frostbite-ramNoFrameskip-v4
Frostbite-v0 Frostbite-v4 FrostbiteDeterministic-v0
FrostbiteDeterministic-v4 FrostbiteNoFrameskip-v0 FrostbiteNoFrameskip-v4
Gopher-ram-v0 Gopher-ram-v4 Gopher-ramDeterministic-v0
Gopher-ramDeterministic-v4 Gopher-ramNoFrameskip-v0 Gopher-ramNoFrameskip-v4
Gopher-v0 Gopher-v4 GopherDeterministic-v0
GopherDeterministic-v4 GopherNoFrameskip-v0 GopherNoFrameskip-v4
Gravitar-ram-v0 Gravitar-ram-v4 Gravitar-ramDeterministic-v0
Gravitar-ramDeterministic-v4 Gravitar-ramNoFrameskip-v0 Gravitar-ramNoFrameskip-v4
Gravitar-v0 Gravitar-v4 GravitarDeterministic-v0
GravitarDeterministic-v4 GravitarNoFrameskip-v0 GravitarNoFrameskip-v4
Hero-ram-v0 Hero-ram-v4 Hero-ramDeterministic-v0
Hero-ramDeterministic-v4 Hero-ramNoFrameskip-v0 Hero-ramNoFrameskip-v4
Hero-v0 Hero-v4 HeroDeterministic-v0
HeroDeterministic-v4 HeroNoFrameskip-v0 HeroNoFrameskip-v4
IceHockey-ram-v0 IceHockey-ram-v4 IceHockey-ramDeterministic-v0
IceHockey-ramDeterministic-v4 IceHockey-ramNoFrameskip-v0 IceHockey-ramNoFrameskip-v4
IceHockey-v0 IceHockey-v4 IceHockeyDeterministic-v0
IceHockeyDeterministic-v4 IceHockeyNoFrameskip-v0 IceHockeyNoFrameskip-v4
Jamesbond-ram-v0 Jamesbond-ram-v4 Jamesbond-ramDeterministic-v0
Jamesbond-ramDeterministic-v4 Jamesbond-ramNoFrameskip-v0 Jamesbond-ramNoFrameskip-v4
Jamesbond-v0 Jamesbond-v4 JamesbondDeterministic-v0
JamesbondDeterministic-v4 JamesbondNoFrameskip-v0 JamesbondNoFrameskip-v4
JourneyEscape-ram-v0 JourneyEscape-ram-v4 JourneyEscape-ramDeterministic-v0
JourneyEscape-ramDeterministic-v4 JourneyEscape-ramNoFrameskip-v0 JourneyEscape-ramNoFrameskip-v4
JourneyEscape-v0 JourneyEscape-v4 JourneyEscapeDeterministic-v0
JourneyEscapeDeterministic-v4 JourneyEscapeNoFrameskip-v0 JourneyEscapeNoFrameskip-v4
Kangaroo-ram-v0 Kangaroo-ram-v4 Kangaroo-ramDeterministic-v0
Kangaroo-ramDeterministic-v4 Kangaroo-ramNoFrameskip-v0 Kangaroo-ramNoFrameskip-v4
Kangaroo-v0 Kangaroo-v4 KangarooDeterministic-v0
KangarooDeterministic-v4 KangarooNoFrameskip-v0 KangarooNoFrameskip-v4
Krull-ram-v0 Krull-ram-v4 Krull-ramDeterministic-v0
Krull-ramDeterministic-v4 Krull-ramNoFrameskip-v0 Krull-ramNoFrameskip-v4
Krull-v0 Krull-v4 KrullDeterministic-v0
KrullDeterministic-v4 KrullNoFrameskip-v0 KrullNoFrameskip-v4
KungFuMaster-ram-v0 KungFuMaster-ram-v4 KungFuMaster-ramDeterministic-v0
KungFuMaster-ramDeterministic-v4 KungFuMaster-ramNoFrameskip-v0 KungFuMaster-ramNoFrameskip-v4
KungFuMaster-v0 KungFuMaster-v4 KungFuMasterDeterministic-v0
KungFuMasterDeterministic-v4 KungFuMasterNoFrameskip-v0 KungFuMasterNoFrameskip-v4
MontezumaRevenge-ram-v0 MontezumaRevenge-ram-v4 MontezumaRevenge-ramDeterministic-v0
MontezumaRevenge-ramDeterministic-v4 MontezumaRevenge-ramNoFrameskip-v0 MontezumaRevenge-ramNoFrameskip-v4
MontezumaRevenge-v0 MontezumaRevenge-v4 MontezumaRevengeDeterministic-v0
MontezumaRevengeDeterministic-v4 MontezumaRevengeNoFrameskip-v0 MontezumaRevengeNoFrameskip-v4
MsPacman-ram-v0 MsPacman-ram-v4 MsPacman-ramDeterministic-v0
MsPacman-ramDeterministic-v4 MsPacman-ramNoFrameskip-v0 MsPacman-ramNoFrameskip-v4
MsPacman-v0 MsPacman-v4 MsPacmanDeterministic-v0
MsPacmanDeterministic-v4 MsPacmanNoFrameskip-v0 MsPacmanNoFrameskip-v4
NameThisGame-ram-v0 NameThisGame-ram-v4 NameThisGame-ramDeterministic-v0
NameThisGame-ramDeterministic-v4 NameThisGame-ramNoFrameskip-v0 NameThisGame-ramNoFrameskip-v4
NameThisGame-v0 NameThisGame-v4 NameThisGameDeterministic-v0
NameThisGameDeterministic-v4 NameThisGameNoFrameskip-v0 NameThisGameNoFrameskip-v4
Phoenix-ram-v0 Phoenix-ram-v4 Phoenix-ramDeterministic-v0
Phoenix-ramDeterministic-v4 Phoenix-ramNoFrameskip-v0 Phoenix-ramNoFrameskip-v4
Phoenix-v0 Phoenix-v4 PhoenixDeterministic-v0
PhoenixDeterministic-v4 PhoenixNoFrameskip-v0 PhoenixNoFrameskip-v4
Pitfall-ram-v0 Pitfall-ram-v4 Pitfall-ramDeterministic-v0
Pitfall-ramDeterministic-v4 Pitfall-ramNoFrameskip-v0 Pitfall-ramNoFrameskip-v4
Pitfall-v0 Pitfall-v4 PitfallDeterministic-v0
PitfallDeterministic-v4 PitfallNoFrameskip-v0 PitfallNoFrameskip-v4
Pong-ram-v0 Pong-ram-v4 Pong-ramDeterministic-v0
Pong-ramDeterministic-v4 Pong-ramNoFrameskip-v0 Pong-ramNoFrameskip-v4
Pong-v0 Pong-v4 PongDeterministic-v0
PongDeterministic-v4 PongNoFrameskip-v0 PongNoFrameskip-v4
Pooyan-ram-v0 Pooyan-ram-v4 Pooyan-ramDeterministic-v0
Pooyan-ramDeterministic-v4 Pooyan-ramNoFrameskip-v0 Pooyan-ramNoFrameskip-v4
Pooyan-v0 Pooyan-v4 PooyanDeterministic-v0
PooyanDeterministic-v4 PooyanNoFrameskip-v0 PooyanNoFrameskip-v4
PrivateEye-ram-v0 PrivateEye-ram-v4 PrivateEye-ramDeterministic-v0
PrivateEye-ramDeterministic-v4 PrivateEye-ramNoFrameskip-v0 PrivateEye-ramNoFrameskip-v4
PrivateEye-v0 PrivateEye-v4 PrivateEyeDeterministic-v0
PrivateEyeDeterministic-v4 PrivateEyeNoFrameskip-v0 PrivateEyeNoFrameskip-v4
Qbert-ram-v0 Qbert-ram-v4 Qbert-ramDeterministic-v0
Qbert-ramDeterministic-v4 Qbert-ramNoFrameskip-v0 Qbert-ramNoFrameskip-v4
Qbert-v0 Qbert-v4 QbertDeterministic-v0
QbertDeterministic-v4 QbertNoFrameskip-v0 QbertNoFrameskip-v4
Riverraid-ram-v0 Riverraid-ram-v4 Riverraid-ramDeterministic-v0
Riverraid-ramDeterministic-v4 Riverraid-ramNoFrameskip-v0 Riverraid-ramNoFrameskip-v4
Riverraid-v0 Riverraid-v4 RiverraidDeterministic-v0
RiverraidDeterministic-v4 RiverraidNoFrameskip-v0 RiverraidNoFrameskip-v4
RoadRunner-ram-v0 RoadRunner-ram-v4 RoadRunner-ramDeterministic-v0
RoadRunner-ramDeterministic-v4 RoadRunner-ramNoFrameskip-v0 RoadRunner-ramNoFrameskip-v4
RoadRunner-v0 RoadRunner-v4 RoadRunnerDeterministic-v0
RoadRunnerDeterministic-v4 RoadRunnerNoFrameskip-v0 RoadRunnerNoFrameskip-v4
Robotank-ram-v0 Robotank-ram-v4 Robotank-ramDeterministic-v0
Robotank-ramDeterministic-v4 Robotank-ramNoFrameskip-v0 Robotank-ramNoFrameskip-v4
Robotank-v0 Robotank-v4 RobotankDeterministic-v0
RobotankDeterministic-v4 RobotankNoFrameskip-v0 RobotankNoFrameskip-v4
Seaquest-ram-v0 Seaquest-ram-v4 Seaquest-ramDeterministic-v0
Seaquest-ramDeterministic-v4 Seaquest-ramNoFrameskip-v0 Seaquest-ramNoFrameskip-v4
Seaquest-v0 Seaquest-v4 SeaquestDeterministic-v0
SeaquestDeterministic-v4 SeaquestNoFrameskip-v0 SeaquestNoFrameskip-v4
Skiing-ram-v0 Skiing-ram-v4 Skiing-ramDeterministic-v0
Skiing-ramDeterministic-v4 Skiing-ramNoFrameskip-v0 Skiing-ramNoFrameskip-v4
Skiing-v0 Skiing-v4 SkiingDeterministic-v0
SkiingDeterministic-v4 SkiingNoFrameskip-v0 SkiingNoFrameskip-v4
Solaris-ram-v0 Solaris-ram-v4 Solaris-ramDeterministic-v0
Solaris-ramDeterministic-v4 Solaris-ramNoFrameskip-v0 Solaris-ramNoFrameskip-v4
Solaris-v0 Solaris-v4 SolarisDeterministic-v0
SolarisDeterministic-v4 SolarisNoFrameskip-v0 SolarisNoFrameskip-v4
SpaceInvaders-ram-v0 SpaceInvaders-ram-v4 SpaceInvaders-ramDeterministic-v0
SpaceInvaders-ramDeterministic-v4 SpaceInvaders-ramNoFrameskip-v0 SpaceInvaders-ramNoFrameskip-v4
SpaceInvaders-v0 SpaceInvaders-v4 SpaceInvadersDeterministic-v0
SpaceInvadersDeterministic-v4 SpaceInvadersNoFrameskip-v0 SpaceInvadersNoFrameskip-v4
StarGunner-ram-v0 StarGunner-ram-v4 StarGunner-ramDeterministic-v0
StarGunner-ramDeterministic-v4 StarGunner-ramNoFrameskip-v0 StarGunner-ramNoFrameskip-v4
StarGunner-v0 StarGunner-v4 StarGunnerDeterministic-v0
StarGunnerDeterministic-v4 StarGunnerNoFrameskip-v0 StarGunnerNoFrameskip-v4
Tennis-ram-v0 Tennis-ram-v4 Tennis-ramDeterministic-v0
Tennis-ramDeterministic-v4 Tennis-ramNoFrameskip-v0 Tennis-ramNoFrameskip-v4
Tennis-v0 Tennis-v4 TennisDeterministic-v0
TennisDeterministic-v4 TennisNoFrameskip-v0 TennisNoFrameskip-v4
TimePilot-ram-v0 TimePilot-ram-v4 TimePilot-ramDeterministic-v0
TimePilot-ramDeterministic-v4 TimePilot-ramNoFrameskip-v0 TimePilot-ramNoFrameskip-v4
TimePilot-v0 TimePilot-v4 TimePilotDeterministic-v0
TimePilotDeterministic-v4 TimePilotNoFrameskip-v0 TimePilotNoFrameskip-v4
Tutankham-ram-v0 Tutankham-ram-v4 Tutankham-ramDeterministic-v0
Tutankham-ramDeterministic-v4 Tutankham-ramNoFrameskip-v0 Tutankham-ramNoFrameskip-v4
Tutankham-v0 Tutankham-v4 TutankhamDeterministic-v0
TutankhamDeterministic-v4 TutankhamNoFrameskip-v0 TutankhamNoFrameskip-v4
UpNDown-ram-v0 UpNDown-ram-v4 UpNDown-ramDeterministic-v0
UpNDown-ramDeterministic-v4 UpNDown-ramNoFrameskip-v0 UpNDown-ramNoFrameskip-v4
UpNDown-v0 UpNDown-v4 UpNDownDeterministic-v0
UpNDownDeterministic-v4 UpNDownNoFrameskip-v0 UpNDownNoFrameskip-v4
Venture-ram-v0 Venture-ram-v4 Venture-ramDeterministic-v0
Venture-ramDeterministic-v4 Venture-ramNoFrameskip-v0 Venture-ramNoFrameskip-v4
Venture-v0 Venture-v4 VentureDeterministic-v0
VentureDeterministic-v4 VentureNoFrameskip-v0 VentureNoFrameskip-v4
VideoPinball-ram-v0 VideoPinball-ram-v4 VideoPinball-ramDeterministic-v0
VideoPinball-ramDeterministic-v4 VideoPinball-ramNoFrameskip-v0 VideoPinball-ramNoFrameskip-v4
VideoPinball-v0 VideoPinball-v4 VideoPinballDeterministic-v0
VideoPinballDeterministic-v4 VideoPinballNoFrameskip-v0 VideoPinballNoFrameskip-v4
WizardOfWor-ram-v0 WizardOfWor-ram-v4 WizardOfWor-ramDeterministic-v0
WizardOfWor-ramDeterministic-v4 WizardOfWor-ramNoFrameskip-v0 WizardOfWor-ramNoFrameskip-v4
WizardOfWor-v0 WizardOfWor-v4 WizardOfWorDeterministic-v0
WizardOfWorDeterministic-v4 WizardOfWorNoFrameskip-v0 WizardOfWorNoFrameskip-v4
YarsRevenge-ram-v0 YarsRevenge-ram-v4 YarsRevenge-ramDeterministic-v0
YarsRevenge-ramDeterministic-v4 YarsRevenge-ramNoFrameskip-v0 YarsRevenge-ramNoFrameskip-v4
YarsRevenge-v0 YarsRevenge-v4 YarsRevengeDeterministic-v0
YarsRevengeDeterministic-v4 YarsRevengeNoFrameskip-v0 YarsRevengeNoFrameskip-v4
Zaxxon-ram-v0 Zaxxon-ram-v4 Zaxxon-ramDeterministic-v0
Zaxxon-ramDeterministic-v4 Zaxxon-ramNoFrameskip-v0 Zaxxon-ramNoFrameskip-v4
Zaxxon-v0 Zaxxon-v4 ZaxxonDeterministic-v0
ZaxxonDeterministic-v4 ZaxxonNoFrameskip-v0 ZaxxonNoFrameskip-v4
===== ALE =====
ALE/Adventure-ram-v5 ALE/Adventure-v5 ALE/AirRaid-ram-v5
ALE/AirRaid-v5 ALE/Alien-ram-v5 ALE/Alien-v5
ALE/Amidar-ram-v5 ALE/Amidar-v5 ALE/Assault-ram-v5
ALE/Assault-v5 ALE/Asterix-ram-v5 ALE/Asterix-v5
ALE/Asteroids-ram-v5 ALE/Asteroids-v5 ALE/Atlantis-ram-v5
ALE/Atlantis-v5 ALE/Atlantis2-ram-v5 ALE/Atlantis2-v5
ALE/Backgammon-ram-v5 ALE/Backgammon-v5 ALE/BankHeist-ram-v5
ALE/BankHeist-v5 ALE/BasicMath-ram-v5 ALE/BasicMath-v5
ALE/BattleZone-ram-v5 ALE/BattleZone-v5 ALE/BeamRider-ram-v5
ALE/BeamRider-v5 ALE/Berzerk-ram-v5 ALE/Berzerk-v5
ALE/Blackjack-ram-v5 ALE/Blackjack-v5 ALE/Bowling-ram-v5
ALE/Bowling-v5 ALE/Boxing-ram-v5 ALE/Boxing-v5
ALE/Breakout-ram-v5 ALE/Breakout-v5 ALE/Carnival-ram-v5
ALE/Carnival-v5 ALE/Casino-ram-v5 ALE/Casino-v5
ALE/Centipede-ram-v5 ALE/Centipede-v5 ALE/ChopperCommand-ram-v5
ALE/ChopperCommand-v5 ALE/Combat-ram-v5 ALE/Combat-v5
ALE/CrazyClimber-ram-v5 ALE/CrazyClimber-v5 ALE/Crossbow-ram-v5
ALE/Crossbow-v5 ALE/Darkchambers-ram-v5 ALE/Darkchambers-v5
ALE/Defender-ram-v5 ALE/Defender-v5 ALE/DemonAttack-ram-v5
ALE/DemonAttack-v5 ALE/DonkeyKong-ram-v5 ALE/DonkeyKong-v5
ALE/DoubleDunk-ram-v5 ALE/DoubleDunk-v5 ALE/Earthworld-ram-v5
ALE/Earthworld-v5 ALE/ElevatorAction-ram-v5 ALE/ElevatorAction-v5
ALE/Enduro-ram-v5 ALE/Enduro-v5 ALE/Entombed-ram-v5
ALE/Entombed-v5 ALE/Et-ram-v5 ALE/Et-v5
ALE/FishingDerby-ram-v5 ALE/FishingDerby-v5 ALE/FlagCapture-ram-v5
ALE/FlagCapture-v5 ALE/Freeway-ram-v5 ALE/Freeway-v5
ALE/Frogger-ram-v5 ALE/Frogger-v5 ALE/Frostbite-ram-v5
ALE/Frostbite-v5 ALE/Galaxian-ram-v5 ALE/Galaxian-v5
ALE/Gopher-ram-v5 ALE/Gopher-v5 ALE/Gravitar-ram-v5
ALE/Gravitar-v5 ALE/Hangman-ram-v5 ALE/Hangman-v5
ALE/HauntedHouse-ram-v5 ALE/HauntedHouse-v5 ALE/Hero-ram-v5
ALE/Hero-v5 ALE/HumanCannonball-ram-v5 ALE/HumanCannonball-v5
ALE/IceHockey-ram-v5 ALE/IceHockey-v5 ALE/Jamesbond-ram-v5
ALE/Jamesbond-v5 ALE/JourneyEscape-ram-v5 ALE/JourneyEscape-v5
ALE/Joust-ram-v5 ALE/Joust-v5 ALE/Kaboom-ram-v5
ALE/Kaboom-v5 ALE/Kangaroo-ram-v5 ALE/Kangaroo-v5
ALE/KeystoneKapers-ram-v5 ALE/KeystoneKapers-v5 ALE/KingKong-ram-v5
ALE/KingKong-v5 ALE/Klax-ram-v5 ALE/Klax-v5
ALE/Koolaid-ram-v5 ALE/Koolaid-v5 ALE/Krull-ram-v5
ALE/Krull-v5 ALE/KungFuMaster-ram-v5 ALE/KungFuMaster-v5
ALE/LaserGates-ram-v5 ALE/LaserGates-v5 ALE/LostLuggage-ram-v5
ALE/LostLuggage-v5 ALE/MarioBros-ram-v5 ALE/MarioBros-v5
ALE/MazeCraze-ram-v5 ALE/MazeCraze-v5 ALE/MiniatureGolf-ram-v5
ALE/MiniatureGolf-v5 ALE/MontezumaRevenge-ram-v5 ALE/MontezumaRevenge-v5
ALE/MrDo-ram-v5 ALE/MrDo-v5 ALE/MsPacman-ram-v5
ALE/MsPacman-v5 ALE/NameThisGame-ram-v5 ALE/NameThisGame-v5
ALE/Othello-ram-v5 ALE/Othello-v5 ALE/Pacman-ram-v5
ALE/Pacman-v5 ALE/Phoenix-ram-v5 ALE/Phoenix-v5
ALE/Pitfall-ram-v5 ALE/Pitfall-v5 ALE/Pitfall2-ram-v5
ALE/Pitfall2-v5 ALE/Pong-ram-v5 ALE/Pong-v5
ALE/Pooyan-ram-v5 ALE/Pooyan-v5 ALE/PrivateEye-ram-v5
ALE/PrivateEye-v5 ALE/Qbert-ram-v5 ALE/Qbert-v5
ALE/Riverraid-ram-v5 ALE/Riverraid-v5 ALE/RoadRunner-ram-v5
ALE/RoadRunner-v5 ALE/Robotank-ram-v5 ALE/Robotank-v5
ALE/Seaquest-ram-v5 ALE/Seaquest-v5 ALE/SirLancelot-ram-v5
ALE/SirLancelot-v5 ALE/Skiing-ram-v5 ALE/Skiing-v5
ALE/Solaris-ram-v5 ALE/Solaris-v5 ALE/SpaceInvaders-ram-v5
ALE/SpaceInvaders-v5 ALE/SpaceWar-ram-v5 ALE/SpaceWar-v5
ALE/StarGunner-ram-v5 ALE/StarGunner-v5 ALE/Superman-ram-v5
ALE/Superman-v5 ALE/Surround-ram-v5 ALE/Surround-v5
ALE/Tennis-ram-v5 ALE/Tennis-v5 ALE/Tetris-ram-v5
ALE/Tetris-v5 ALE/TicTacToe3D-ram-v5 ALE/TicTacToe3D-v5
ALE/TimePilot-ram-v5 ALE/TimePilot-v5 ALE/Trondead-ram-v5
ALE/Trondead-v5 ALE/Turmoil-ram-v5 ALE/Turmoil-v5
ALE/Tutankham-ram-v5 ALE/Tutankham-v5 ALE/UpNDown-ram-v5
ALE/UpNDown-v5 ALE/Venture-ram-v5 ALE/Venture-v5
ALE/VideoCheckers-ram-v5 ALE/VideoCheckers-v5 ALE/VideoChess-ram-v5
ALE/VideoChess-v5 ALE/VideoCube-ram-v5 ALE/VideoCube-v5
ALE/VideoPinball-ram-v5 ALE/VideoPinball-v5 ALE/Warlords-ram-v5
ALE/Warlords-v5 ALE/WizardOfWor-ram-v5 ALE/WizardOfWor-v5
ALE/WordZapper-ram-v5 ALE/WordZapper-v5 ALE/YarsRevenge-ram-v5
ALE/YarsRevenge-v5 ALE/Zaxxon-ram-v5 ALE/Zaxxon-v5
===== rldurham =====
rldurham/Walker
Total running time of the script: (0 minutes 7.873 seconds)